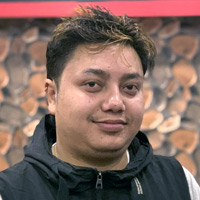
Suman Awal
January 7, 2025
Rails 8 comes with the built-in functionality for the User authentication. However, it does not have user registration. Here, we will create a simple Rails application with the complete user registration, authentication, and destroy session features.
Ensure that Rails 8 is installed on the machine before starting.
- Create a Rails Application
rails new authentication_with_rails_8 --css=tailwind
- Generate rails authentication
rails g authentication
app/models/current.rb app/models/user.rb app/models/session.rb app/controllers/sessions_controller.rb app/controllers/passwords_controller.rb app/mailers/passwords_mailer.rb app/views/sessions/new.html.erb app/views/passwords/new.html.erb app/views/passwords/edit.html.erb app/views/passwords_mailer/reset.html.erb app/views/passwords_mailer/reset.text.erb db/migrate/xxxxxxx_create_users.rb db/migrate/xxxxxxx_create_sessions.rb test/mailers/previews/passwords_mailer_preview.rb
- User Registration
With the rails generation above it does not provide the functionality of User registration. Now let's create a user registration functionality.
-
Create a registrations controller
# app/controllers/registrations_controller.rb class RegistrationsController < ApplicationController allow_unauthenticated_access def new @user = User.new end def create @user = User.new(user_params) if @user.save start_new_session_for @user redirect_to root_path, notice: 'User successfully signed up.' else flash[:alert] = @user.errors.full_messages.join("\n") render :new end end private def user_params params.require(:user).permit(:email_address, :password, :password_confirmation) end end
- Update routes Add routes for the registrations controller and a root path to navigate after signup/login.
# config/routes # Add the following ling resources registrations, only: [:new, :create] root "home#index"
- Create views Create a form for the user registration.
# app/views/registrations/new.html.erb
<% if alert = flash[:alert] %><%= alert %>
<% end %>Sign up
<%= form_with model: @user, url: registrations_url, class: "contents" do |form| %><%= form.email_field :email_address, required: true, autofocus: true, value: params[:email_address], class: "block shadow rounded-md border border-gray-400 px-3 py-2 mt-2 w-full" %><%= form.password_field :password, required: true, placeholder: "Enter your password", maxlength: 72, class: "block shadow rounded-md border border-gray-400 px-3 py-2 mt-2 w-full" %><%= form.password_field :password_confirmation, required: true, placeholder: "Confirm your password", maxlength: 72, class: "block shadow rounded-md border border-gray-400 px-3 py-2 mt-2 w-full" %><%= form.submit "Sign up", class: "rounded-lg py-3 px-5 bg-blue-600 text-white inline-block cursor-pointer" %>
-
Create a registrations controller
- Create a home page to navigate after login.
- Create controller
# app/controllers/home_controller.rb class HomeController < ApplicationController def index; end end
- Create Views
# app/views/home/index.html.erb <%= link_to 'Logout', session_path, method: :delete, data: {turbo_method: :delete} %>
- Create controller
Conclusion
In this way, we can create a complete authentication system with the built-in feature provided by Rails 8.
Ps. if you have any questions
Ask here