Merge Hashes for simplified data handling
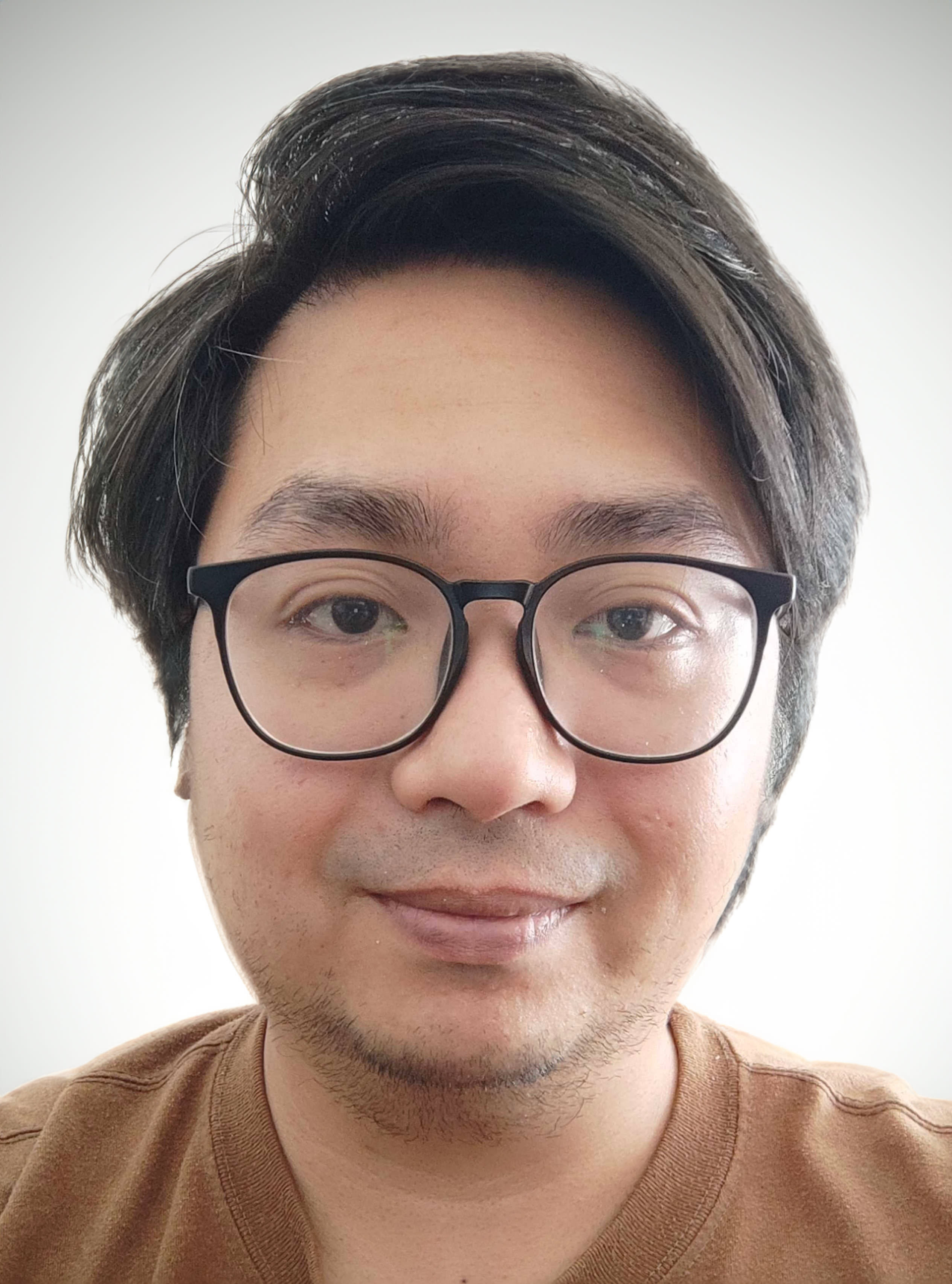
As Ruby on Rails developers, we would like to effectively handle cases with the right tools in hand. One of the key methods that Ruby offers is .merge. This method combines the content of two hashes.
You can find the documentation of .merge in here
Handling Data
This allows developers to combine data from different sources into one structure. One example is dealing with parameters in a controller. Merging the params makes all information accessible in one variable.
combine_params = params.merge(additional_data)
Code Readability
Instead of using multiple lines of code to add or update key-value pairs, .merge does it in one go.
# Instead of this
params[:key1] = value1
params[:key2] = value2
# We can use this
params.merge!(key1: value1, key2: value2)
Handle Overwriting
When provided with a block, merge allows you to customize handling of the instances that the keys were duplicated. It uses the values returned within the block.
h1 = { a:1, b:2 }
h2 = { b:3, c:10 }
new_hash = h1.merge(h2) { |key, old_value, new_value| old_value + new_value }
new_hash
{ a: 1, b:5, c:10 }
Conclusion
Ruby offers different methods that allows developers to be more effective with their code. .merge is certainly used in many codebases and will be used further in future Rails projects.