Array operations using Ruby
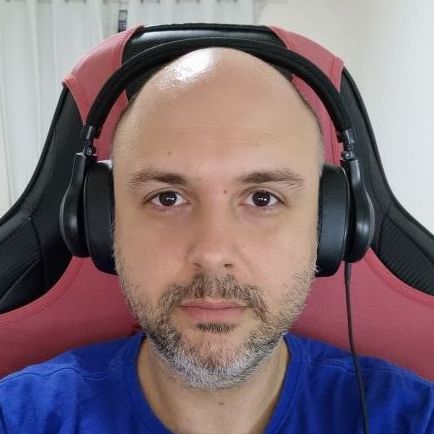
Getting your code clean and readable is one of the most satisfying things for Rubist. Today, I’ll share with you some good methods to make your code shine when working with arrays.
Array#intersection
This method was first introduced in the Ruby 3.1 release. It takes the same path of Array#difference
and Array#union
to have a more clear alias. Another way to call it is Array#&
. As a set operation, a good thing to notice about this method is the result of the operation keeps the order of the original array as the resultant array has unique values.
[10,20,30,40,50].intersection([30,10,60,75])
=> [10, 30]
You can also pass multiple arrays as parameters:
[ "1", "2", "3" ].intersection([ "a", "1", "d" ], ['1'])
=> [ "1" ]
Array#intersect?
This method allows you to check if the arrays contain elements in common.
[10,20,30,40,50].intersect?([30,10,60,75])
=> true
Array#difference
Can you guess this one? :) This method returns a new array that contains only the items that do not appear in the context (the original array) and the arguments.
[10,20,30,40,50].difference([30,10,60,75])
=> [20, 40, 50]
As with the others, multiple arrays can be used as arguments of the method:
[ 2, 3, 1, "a", :j ].difference([ 7, :t ], [ "2" ])
=> [2, 3, 1, "a", :j]
Array#union
The opposite of the Array#difference
method, Array#union
returns a new array that has all the items of the other arrays, removing the possible duplicity of items.
It’s a good choice for this type of situation:
Ok option
[1,2,3].concat([2,3,4]).uniq
=> [1, 2, 3, 4]
Shine option
[1,2,3].union([2,3,4])
=> [1, 2, 3, 4]
Do you know any other cool Array methods? Share it with us!
That’s it for today! See you all next post!