Ruby: Everything Is An Object?
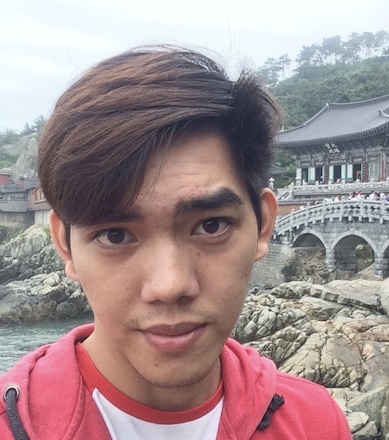
When you were learning ruby, you might have encountered that everything in ruby is an object. But is it really though? To find the answer, we have to define what an object is; but before that, we have to know first what is a class.
A class is like a blueprint of anything, you (as a developer) provide its properties and its behavior. See below as an example:
class Vehicle
attr_accessor :color, :maker, :fuel_amount
def accelerate
# insert code here to define class behavior
end
def stop
# or here
end
end
Above is an overly-simplified Vehicle
class with three properties/attributes and two methods. It’s entirely up to you to define its behavior. You can make the vehicle be able to accelerate if and only if, when the value of its color
property is blue. Realistically, the vehicle should only be able to accelerate if it has fuel, i.e. fuel_amount > 0
. It is important to note that this class’ properties have no specific values yet; you’re just merely defining their existence.
An object is the end-product after realizing what’s in the blueprint, i.e. the class. Its color
can either be red or any other color. Its fuel_amount
can either be empty, or full, or any amount in between.
toyota = Vehicle.new
toyota.color = 'green'
toyota.maker = 'toyota'
toyota.fuel_amount = 25
honda = Vehicle.new
honda.color = 'purple'
honda.maker = 'honda'
In above example, we created two objects from the Vehicle
class, referenced by the two variables: toyota
and honda
. In other words, we created two instances of Vehicle
or instantiated it twice. So what the code above says is that toyota
is a green Toyota vehicle with a quarter amount of fuel, while honda
is a purple Honda vehicle with a full amount of fuel.
How about the values that were assigned to these two objects’ properties? Are they objects as well? In ruby, yes they are! Those values that are enclosed in single quotation marks, e.g. 'green'
, 'honda'
, are instances of the String
class, while the numerical values are instances of Integer
. In fact, even the Vehicle
class defined earlier, is an instance of Class
class. Therefore, even classes are objects! You can verify it below:
Vehicle.class.name
=> "Class"
How about the accelerate
and stop
methods defined in the Vehicle
class? While there is a Method
class defined in ruby, these two methods aren’t instances of the Method
class. These methods are two separate collections of codes/statements which are invoked when a method is called. You can verify it below:
toyota.accelerate.class.name
=> "NilClass"
In above code, calling the accelerate
method of the earlier toyota
object does not give us an instance of a Method
class but of NilClass
which is the class of the return value, after invoking the collection of statements of this method (which in this example is blank or nil
).
However, in ruby, you can still reference the methods defined in your class, as instances of the Method
class like below:
toyota.method(:accelerate)
=> #<Method: Vehicle#accelerate()>
toyota.method(:accelerate).class.name
=> "Method"
How about earlier when we instantiated the Vehicle
class and assigned them to these two variables: toyota
and honda
? We know that the values of these variables are instances of the Vehicle
class but what about the variables themselves? Variables aren’t objects; they’re just references/identifiers to the objects and not the objects themselves.
Another one are blocks, which are almost the same with methods and also have object counterparts, called procs or lambdas.
So, to answer the question - is everything an object in ruby? Well, technically it’s a no but every value that a property or a method returns is an object.