Front-End Testing in Rails with Capybara and RSpec
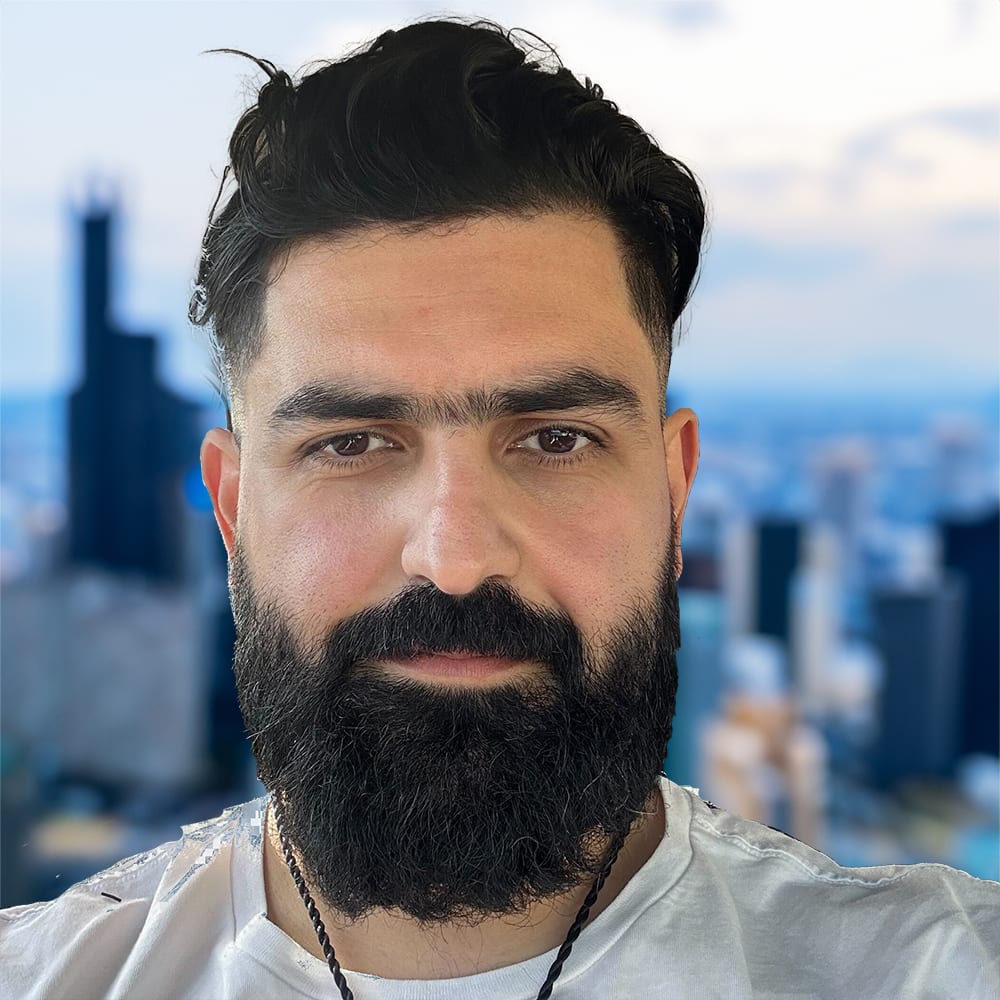
Front-end testing is a critical part of web application development, ensuring that your user interface functions correctly and delivers a seamless experience. In the Ruby on Rails ecosystem, Capybara and RSpec are powerful tools for writing comprehensive front-end tests. In this blog post, we’ll explore front-end testing with Capybara and RSpec, starting with a simple example and progressing to a more complex one.
Setting Up Capybara and RSpec:
Before we dive into testing, you’ll need to set up Capybara and RSpec in your Rails project. Begin by adding the following gems to your Gemfile
:
group :test do
gem 'capybara'
gem 'rspec-rails'
end
After adding the gems, run bundle install
to install them. Next, generate RSpec configuration with:
rails generate rspec:install
Simple Example: Testing User Login: Let’s start with a simple example by testing a user login page. Consider a Rails application with a basic login feature. You can create a test for it like this:
# spec/features/user_login_spec.rb
require 'rails_helper'
describe 'User Login' do
it 'allows a user to log in' do
visit '/login'
fill_in 'Email', with: 'user@example.com'
fill_in 'Password', with: 'password'
click_button 'Log in'
expect(page).to have_content('Welcome, user!')
end
end
This test visits the login page, enters login credentials, and verifies the presence of a welcome message.
Complex Example: Testing a Multi-Step Form: Now, let’s explore a more complex example involving a multi-step registration form. Suppose you have a multi-step registration process in your application. To test it, you can create a feature spec like this:
# spec/features/user_registration_spec.rb
require 'rails_helper'
describe 'User Registration' do
it 'successfully completes the multi-step registration form' do
visit '/registration' # Starting point
# Step 1: Fill in user information
fill_in 'First Name', with: 'John'
fill_in 'Last Name', with: 'Doe'
click_button 'Next'
# Step 2: Fill in email and password
fill_in 'Email', with: 'john@example.com'
fill_in 'Password', with: 'secure_password'
click_button 'Next'
# Step 3: Verify the final step and submit
expect(page).to have_content('Review your information')
click_button 'Complete Registration'
# Check for a successful registration message
expect(page).to have_content('Registration successful!')
end
end
This test simulates a user’s journey through a multi-step registration form, ensuring a successful registration and the display of a registration success message.
Comparing Automated Testing with Other Methods: While automated front-end testing with tools like Capybara and RSpec provides many advantages, it’s essential to consider how it compares to other testing methods, such as Rails system tests and Selenium.
1. Rails System Tests: Rails introduced system tests with the introduction of system testing in Rails 5.1. System tests are higher-level tests that simulate user interactions. They use Capybara under the hood but are more integrated with the Rails testing framework. They provide a way to test your application in a more Rails-specific context.
Advantages:
- Tight integration with Rails.
- Simplified setup and configuration.
2. Selenium: Selenium is a widely-used browser automation tool that can be used in combination with Capybara for front-end testing. Selenium offers broad browser support and a wide range of testing capabilities, making it a robust choice for complex testing scenarios.
Advantages:
- Cross-browser compatibility testing.
- Extensive test capabilities for complex scenarios.
Comparing Automated Testing with Manual Testing: When deciding between automated testing and manual testing, it’s crucial to understand the differences and choose the approach that best suits your project’s needs.
Automated Testing:
- Provides consistency and repeatability in testing scenarios.
- Allows for testing a wide range of user interactions and edge cases.
- Speeds up the testing process, especially in large and complex applications.
- Easily integrates into your development environment and continuous integration processes, catching issues early.
Manual Testing:
- Offers the human touch to identify usability and design issues that automated tests might miss.
- Is well-suited for exploratory testing and user experience evaluation.
- Is more flexible for ad-hoc testing but can be time-consuming and error-prone.
- Tends to be less efficient and suitable for repetitive tasks.
Conclusion: We’ve covered front-end testing in Rails using Capybara and RSpec, with both simple and complex examples. Capybara and RSpec offer a flexible and effective testing solution for Rails applications.
When deciding between automated testing and manual testing, consider your project’s requirements and constraints. Automated testing with Capybara and RSpec can significantly enhance your testing process, providing consistent, repeatable, and efficient testing, especially for complex applications. Manual testing, on the other hand, remains valuable for exploring usability and design aspects that automation cannot capture.
Ultimately, the choice between automated and manual testing should be based on your project’s specific needs and goals.
Thanks for reading, no matter your software problem, we provide a complete solution to scope, design, develop, test, host, maintain and support it 24/7/365. Contact us to find out how can we bring your ideas to reality!